Catching Crypto Secrets in Android Apps with Frida
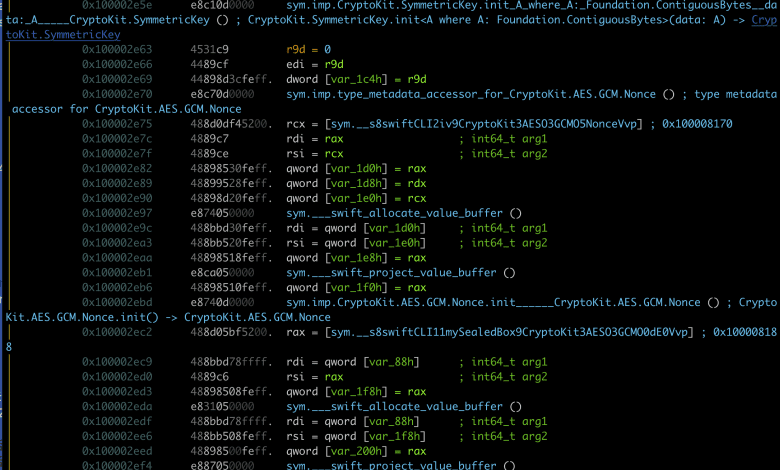
You know that feeling when you’re staring at an Android app and wondering “what the hell is this thing doing with my data?” Well, today we’re going to pull back the curtain and see exactly how apps handle encryption, hashing, and all that crypto stuff that usually happens in the shadows.
Why This Matters
Think about it – every time you log into an app, make a payment, or send a message, there’s crypto happening behind the scenes. Some apps do it right, others… well, let’s just say they get creative in ways that would make security experts cry.
Whether you’re a pentester trying to find weak spots, a developer debugging your own app, or just someone who’s curious about what’s really going on under the hood, this guide will show you how to catch every single crypto operation an Android app makes.
Setting Up Your Lab
Before we dive in, let’s get everything ready. You’ll need a proper setup to make this work smoothly.
The Basics
First, make sure you’ve got Frida installed properly:
1 2 |
pip install frida-tools |
You’ll also need a rooted Android device or an emulator. If you’re using an emulator, I recommend using something like Genymotion or the Android Studio emulator with a rooted image.
For the device setup:
1 2 3 4 5 6 7 8 |
# Check if Frida server is running on your device adb shell ps | grep frida # If not, push and run frida-server adb push frida-server /data/local/tmp/ adb shell "chmod 755 /data/local/tmp/frida-server" adb shell "/data/local/tmp/frida-server &" |
Finding Your Target
List all the apps running on your device:
1 2 |
frida-ps -U |
Or if you want to see all installed apps:
1 2 |
frida-ps -Ua |
Understanding the Crypto Landscape
Android apps primarily use Java’s crypto APIs, which means they’re calling methods from packages like:
javax.crypto.*
– For encryption, decryption, key generationjava.security.*
– For hashing, signatures, key managementandroid.security.keystore.*
– For hardware-backed key operations
Our script hooks into all of these, giving us a complete picture of what’s happening.
The Complete Tracing Script
Here’s the full script that does the heavy lifting. Save this as crypto_tracer.js
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 |
function bin2ascii(array) { var result = []; for (var i = 0; i < array.length; ++i) { result.push(String.fromCharCode( parseInt( ('0' + (array[i] & 0xFF).toString(16)).slice(-2), 16 ) )); } return result.join(''); } function bin2hex(array, length) { var result = ""; length = length || array.length; for (var i = 0; i < length; ++i) { result += ('0' + (array[i] & 0xFF).toString(16)).slice(-2); } return result; } setTimeout(function() { Java.perform(function() { console.log("[+] Crypto tracer started - hooking into crypto operations..."); var Log = Java.use("android.util.Log") var Exception = Java.use("java.lang.Exception") // Hook KeyGenerator operations var keyGenerator = Java.use("javax.crypto.KeyGenerator"); keyGenerator.generateKey.implementation = function() { console.log("[🔑] KeyGenerator.generateKey() called"); console.log(" Algorithm: " + this.getAlgorithm()); var result = this.generateKey(); console.log(" Key generated successfully"); return result; }; keyGenerator.getInstance.overload('java.lang.String').implementation = function(algorithm) { console.log("[🔑] KeyGenerator.getInstance() called"); console.log(" Algorithm: " + algorithm); return this.getInstance(algorithm); }; keyGenerator.getInstance.overload('java.lang.String', 'java.lang.String').implementation = function(algorithm, provider) { console.log("[🔑] KeyGenerator.getInstance() called"); console.log(" Algorithm: " + algorithm); console.log(" Provider: " + provider); return this.getInstance(algorithm, provider); }; // Hook SecretKeySpec - this is where we see actual key material var secretKeySpec = Java.use("javax.crypto.spec.SecretKeySpec"); secretKeySpec.$init.overload('[B', 'java.lang.String').implementation = function(keyBytes, algorithm) { var key = Java.array('byte', keyBytes); console.log("[🗝️ ] SecretKeySpec created:"); console.log(" Algorithm: " + algorithm); console.log(" Key (hex): " + bin2hex(key)); console.log(" Key (ascii): " + bin2ascii(key)); console.log(" Key length: " + key.length + " bytes"); return secretKeySpec.$init.overload('[B', 'java.lang.String').call(this, keyBytes, algorithm); } // Hook Cipher operations var cipher = Java.use("javax.crypto.Cipher"); cipher.getInstance.overload('java.lang.String').implementation = function(transformation) { console.log("[🔐] Cipher.getInstance() called"); console.log(" Transformation: " + transformation); return this.getInstance(transformation); }; cipher.init.overload('int', 'java.security.Key').implementation = function(opmode, key) { var mode = opmode == 1 ? "ENCRYPT" : opmode == 2 ? "DECRYPT" : "UNKNOWN(" + opmode + ")"; console.log("[🔐] Cipher.init() called"); console.log(" Mode: " + mode); console.log(" Algorithm: " + this.getAlgorithm()); return this.init(opmode, key); }; cipher.init.overload('int', 'java.security.Key', 'java.security.spec.AlgorithmParameterSpec').implementation = function(opmode, key, params) { var mode = opmode == 1 ? "ENCRYPT" : opmode == 2 ? "DECRYPT" : "UNKNOWN(" + opmode + ")"; console.log("[🔐] Cipher.init() called (with params)"); console.log(" Mode: " + mode); console.log(" Algorithm: " + this.getAlgorithm()); console.log(" Params: " + params); return this.init(opmode, key, params); }; cipher.doFinal.overload('[B').implementation = function(input) { console.log("[🔐] Cipher.doFinal() called"); console.log(" Input length: " + input.length + " bytes"); console.log(" Input (hex): " + bin2hex(Java.array('byte', input))); var result = cipher.doFinal.overload("[B").call(this, input); console.log(" Output length: " + result.length + " bytes"); console.log(" Output (hex): " + bin2hex(Java.array('byte', result))); return result; }; // Hook MessageDigest (hashing) var messageDigest = Java.use("java.security.MessageDigest"); messageDigest.getInstance.overload('java.lang.String').implementation = function(algorithm) { console.log("[🔒] MessageDigest.getInstance() called"); console.log(" Algorithm: " + algorithm); return this.getInstance(algorithm); }; messageDigest.digest.overload().implementation = function() { console.log("[🔒] MessageDigest.digest() called"); console.log(" Algorithm: " + this.getAlgorithm()); var result = messageDigest.digest.overload().call(this); var hash = Java.array('byte', result); console.log(" Hash (hex): " + bin2hex(hash)); console.log(" Hash length: " + hash.length + " bytes"); return result; }; messageDigest.digest.overload('[B').implementation = function(input) { console.log("[🔒] MessageDigest.digest() called with input"); console.log(" Algorithm: " + this.getAlgorithm()); console.log(" Input length: " + input.length + " bytes"); console.log(" Input (hex): " + bin2hex(Java.array('byte', input))); var result = messageDigest.digest.overload('[B').call(this, input); var hash = Java.array('byte', result); console.log(" Hash (hex): " + bin2hex(hash)); return result; }; // Hook Mac (HMAC operations) var mac = Java.use("javax.crypto.Mac"); mac.getInstance.overload('java.lang.String').implementation = function(algorithm) { console.log("[🔐] Mac.getInstance() called"); console.log(" Algorithm: " + algorithm); return this.getInstance(algorithm); }; mac.init.overload('java.security.Key').implementation = function(key) { console.log("[🔐] Mac.init() called"); console.log(" Algorithm: " + this.getAlgorithm()); return this.init(key); }; mac.doFinal.overload().implementation = function() { console.log("[🔐] Mac.doFinal() called"); console.log(" Algorithm: " + this.getAlgorithm()); var result = mac.doFinal.overload().call(this); var hmac = Java.array('byte', result); console.log(" HMAC (hex): " + bin2hex(hmac)); console.log(" HMAC length: " + hmac.length + " bytes"); return result; }; // Hook Android Keystore operations var keyStore = Java.use("java.security.KeyStore"); keyStore.getInstance.overload('java.lang.String').implementation = function(type) { console.log("[🏪] KeyStore.getInstance() called"); console.log(" Type: " + type); return this.getInstance(type); }; keyStore.load.overload('java.io.InputStream', '[C').implementation = function(stream, password) { console.log("[🏪] KeyStore.load() called"); if (password) { console.log(" Password length: " + password.length + " chars"); } return this.load(stream, password); }; // Hook KeyGenParameterSpec for hardware-backed keys try { var keyGenBuilder = Java.use("android.security.keystore.KeyGenParameterSpec$Builder"); keyGenBuilder.$init.overload("java.lang.String", "int").implementation = function(keystoreAlias, purposes) { var purposeStr = ""; if (purposes & 1) purposeStr += "ENCRYPT "; if (purposes & 2) purposeStr += "DECRYPT "; if (purposes & 4) purposeStr += "SIGN "; if (purposes & 8) purposeStr += "VERIFY "; console.log("[🔐] KeyGenParameterSpec.Builder created"); console.log(" Keystore alias: " + keystoreAlias); console.log(" Purposes: " + purposeStr + "(" + purposes + ")"); return keyGenBuilder.$init.overload("java.lang.String", "int").call(this, keystoreAlias, purposes); }; keyGenBuilder.setKeySize.implementation = function(keySize) { console.log("[🔐] KeyGenParameterSpec: Key size set to " + keySize + " bits"); return this.setKeySize(keySize); }; keyGenBuilder.setBlockModes.implementation = function(blockModes) { console.log("[🔐] KeyGenParameterSpec: Block modes: " + blockModes); return this.setBlockModes(blockModes); }; keyGenBuilder.setEncryptionPaddings.implementation = function(paddings) { console.log("[🔐] KeyGenParameterSpec: Encryption paddings: " + paddings); return this.setEncryptionPaddings(paddings); }; } catch(e) { console.log("[!] KeyGenParameterSpec hooking failed (Android version might not support it)"); } // Hook IvParameterSpec to catch initialization vectors var ivSpec = Java.use("javax.crypto.spec.IvParameterSpec"); ivSpec.$init.overload("[B").implementation = function(iv) { var ivArray = Java.array('byte', iv); console.log("[🔒] IvParameterSpec created"); console.log(" IV (hex): " + bin2hex(ivArray)); console.log(" IV (ascii): " + bin2ascii(ivArray)); console.log(" IV length: " + ivArray.length + " bytes"); return ivSpec.$init.overload("[B").call(this, iv); }; // Hook Signature operations var signature = Java.use("java.security.Signature"); signature.getInstance.overload('java.lang.String').implementation = function(algorithm) { console.log("[✍️] Signature.getInstance() called"); console.log(" Algorithm: " + algorithm); return this.getInstance(algorithm); }; signature.initSign.overload('java.security.PrivateKey').implementation = function(privateKey) { console.log("[✍️] Signature.initSign() called"); console.log(" Algorithm: " + this.getAlgorithm()); return this.initSign(privateKey); }; signature.initVerify.overload('java.security.PublicKey').implementation = function(publicKey) { console.log("[✍️] Signature.initVerify() called"); console.log(" Algorithm: " + this.getAlgorithm()); return this.initVerify(publicKey); }; signature.sign.overload().implementation = function() { console.log("[✍️] Signature.sign() called"); console.log(" Algorithm: " + this.getAlgorithm()); var result = signature.sign.overload().call(this); var sig = Java.array('byte', result); console.log(" Signature (hex): " + bin2hex(sig)); console.log(" Signature length: " + sig.length + " bytes"); return result; }; console.log("[+] All crypto hooks installed successfully!"); console.log("[+] Waiting for crypto operations..."); }); }, 0); |
Running the Tracer
Now that you have the script, here’s how to use it:
Basic Usage
1 2 3 4 5 6 |
# Attach to a running app frida -U -l crypto_tracer.js com.example.app # Or spawn a new instance of the app frida -U -f com.example.app -l crypto_tracer.js --no-pause |
Advanced Usage with Output Logging
If you want to save everything to a file:
1 2 |
frida -U -l crypto_tracer.js com.example.app | tee crypto_trace.log |
Or if you want to filter only specific operations:
1 2 |
frida -U -l crypto_tracer.js com.example.app | grep -E "\[🔑\]|\[🔐\]" |
Understanding the Output
When you run the tracer, you’ll see output like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
[+] Crypto tracer started - hooking into crypto operations... [🔑] KeyGenerator.getInstance() called Algorithm: AES [🔑] KeyGenerator.generateKey() called Algorithm: AES Key generated successfully [🗝️ ] SecretKeySpec created: Algorithm: AES Key (hex): 48656c6c6f576f726c6448656c6c6f Key (ascii): HelloWorldHello Key length: 16 bytes [🔐] Cipher.getInstance() called Transformation: AES/CBC/PKCS5Padding [🔒] IvParameterSpec created IV (hex): 0102030405060708090a0b0c0d0e0f10 IV (ascii): ................ IV length: 16 bytes [🔐] Cipher.init() called (with params) Mode: ENCRYPT Algorithm: AES/CBC/PKCS5Padding Params: javax.crypto.spec.IvParameterSpec@a1b2c3d4 [🔐] Cipher.doFinal() called Input length: 32 bytes Input (hex): 54686973206973206120736563726574206d6573736167652074686174206e6565647320656e6372797074696f6e Output length: 48 bytes Output (hex): 3f8a9c2b1e4d6f7a8b3c9e1f2a4b5c6d7e8f9a0b1c2d3e4f5a6b7c8d9e0f1a2b |
What Each Symbol Means
- 🔑 KeyGenerator operations – When the app generates new encryption keys
- 🗝️ SecretKeySpec – Shows you the actual key material being used
- 🔐 Cipher operations – Encryption/decryption activities with full details
- 🔒 MessageDigest/IV – Hashing operations and initialization vectors
- ✍️ Signature operations – Digital signing and verification
- 🏪 KeyStore operations – When the app uses Android’s secure key storage
Real-World Analysis Examples
Example 1: Banking App Analysis
Let’s say you’re analyzing a banking app. You might see:
1 2 |
frida -U -l crypto_tracer.js com.bank.app |
Look for patterns like:
- Are they using AES with proper key lengths (256-bit)?
- Do they use random IVs for each encryption?
- Are passwords being hashed with salt?
- Are they using hardware-backed keys for sensitive operations?
Example 2: Messaging App Research
For a messaging app, you’d want to check:
1 2 |
frida -U -f com.chat.app -l crypto_tracer.js --no-pause |
Key things to look for:
- End-to-end encryption implementation
- Key exchange mechanisms
- Message signing for authenticity
Example 3: Custom Analysis Script
You can also create a custom analysis script that builds on our tracer:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
// analysis.js - Custom script for specific app analysis Java.perform(function() { // Load the base tracer first // Then add custom logic var suspicious_keys = []; var crypto_operations = []; // Override our SecretKeySpec hook to collect keys var originalSecretKeySpec = secretKeySpec.$init.overload('[B', 'java.lang.String').implementation; secretKeySpec.$init.overload('[B', 'java.lang.String').implementation = function(keyBytes, algorithm) { var key = Java.array('byte', keyBytes); var keyHex = bin2hex(key); // Check for weak keys (all zeros, repeated patterns, etc.) if (keyHex.match(/^(00)+$/)) { console.log("[⚠️ ] WARNING: All-zero key detected!"); suspicious_keys.push({type: "zero_key", key: keyHex, algorithm: algorithm}); } if (keyHex.match(/^(.{2})\1+$/)) { console.log("[⚠️ ] WARNING: Repeating pattern in key detected!"); suspicious_keys.push({type: "pattern_key", key: keyHex, algorithm: algorithm}); } crypto_operations.push({ type: "key_creation", algorithm: algorithm, key: keyHex, timestamp: new Date().toISOString() }); return originalSecretKeySpec.call(this, keyBytes, algorithm); }; // Export findings after 30 seconds setTimeout(function() { console.log("\n=== ANALYSIS SUMMARY ==="); console.log("Total crypto operations: " + crypto_operations.length); console.log("Suspicious keys found: " + suspicious_keys.length); if (suspicious_keys.length > 0) { console.log("\nSUSPICIOUS KEYS:"); suspicious_keys.forEach(function(key, index) { console.log((index + 1) + ". " + key.type + " - " + key.algorithm + " - " + key.key); }); } // You could also export this data to a file or send it to a server }, 30000); }); |
Advanced Techniques
Bypassing Anti-Frida Measures
Some apps try to detect Frida. Here’s how to deal with that:
1 2 3 4 5 6 7 8 |
# Rename frida-server to something innocent adb shell "mv /data/local/tmp/frida-server /data/local/tmp/system_daemon" adb shell "/data/local/tmp/system_daemon &" # Use a different port adb shell "/data/local/tmp/frida-server -l 0.0.0.0:8888 &" frida -H 127.0.0.1:8888 -l crypto_tracer.js com.example.app |
Automated Analysis with Python
You can also automate the analysis with Python:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
import frida import sys import json import time def on_message(message, data): if message['type'] == 'send': payload = message['payload'] if 'SecretKeySpec' in payload: print(f"[KEY DETECTED] {payload}") elif 'Cipher.doFinal' in payload: print(f"[CRYPTO OP] {payload}") device = frida.get_usb_device() session = device.attach("com.example.app") with open("crypto_tracer.js", "r") as f: script_content = f.read() script = session.create_script(script_content) script.on('message', on_message) script.load() print("[*] Script loaded, press Ctrl+C to exit") try: sys.stdin.read() except KeyboardInterrupt: print("\n[*] Stopping...") session.detach() |
Extracting and Saving Key Material
Want to save all the key material for later analysis? Modify the script:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Add this to the SecretKeySpec hook var fs = Java.use("java.io.FileOutputStream"); var keyFile = "/sdcard/Download/extracted_keys.txt"; var keyData = algorithm + "|" + bin2hex(key) + "|" + new Date().toISOString() + "\n"; try { var fos = fs.$new(keyFile, true); // true for append mode var bytes = Java.array('byte', keyData.split('').map(c => c.charCodeAt(0))); fos.write(bytes); fos.close(); console.log(" Key saved to: " + keyFile); } catch(e) { console.log(" Failed to save key: " + e); } |
Interpreting Common Patterns
Good Crypto Practices
When you see these patterns, it usually means the app is doing things right:
1 2 3 4 5 6 7 8 9 |
[🔑] KeyGenerator.getInstance() called Algorithm: AES [🗝️ ] SecretKeySpec created: Algorithm: AES Key length: 32 bytes # 256-bit key - good! [🔒] IvParameterSpec created IV length: 16 bytes # Proper IV size for AES IV (hex): a1b2c3d4e5f6... # Random-looking IV - good! |
Red Flags
Watch out for these warning signs:
1 2 3 4 5 |
[🗝️ ] SecretKeySpec created: Algorithm: DES # DES is broken! Key (hex): 0000000000000000 # All-zero key! Key (ascii): HelloWorld # Hardcoded key! |
1 2 3 |
[🔒] MessageDigest.getInstance() called Algorithm: MD5 # MD5 is broken for crypto! |
1 2 3 |
[🔒] IvParameterSpec created IV (hex): 0102030405060708090a0b0c0d0e0f10 # Static IV - bad! |
Building Your Own Crypto Arsenal
Monitoring Specific Algorithms
If you want to focus on specific algorithms, create a targeted script:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Focus only on AES operations Java.perform(function() { // ... (previous code) var originalCipherGetInstance = cipher.getInstance.overload('java.lang.String').implementation; cipher.getInstance.overload('java.lang.String').implementation = function(transformation) { if (transformation.toLowerCase().includes('aes')) { console.log("[🎯] AES operation detected: " + transformation); // You could trigger additional analysis here // Maybe dump the stack trace to see where it's called from console.log("Stack trace: " + Log.getStackTraceString(Exception.$new())); } return originalCipherGetInstance.call(this, transformation); }; }); |
Real-Time Key Analysis
Want to analyze keys as they’re created?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// Add to SecretKeySpec hook var keyHex = bin2hex(key); var keyStrength = analyzeKeyStrength(keyHex); function analyzeKeyStrength(hexKey) { var analysis = { length: hexKey.length / 2, entropy: calculateEntropy(hexKey), patterns: findPatterns(hexKey), strength: "unknown" }; if (analysis.entropy < 3.0) { analysis.strength = "weak"; console.log(" [⚠️ ] LOW ENTROPY KEY DETECTED!"); } else if (analysis.entropy > 7.0) { analysis.strength = "strong"; console.log(" [✅] Good entropy detected"); } return analysis; } function calculateEntropy(hexString) { var frequency = {}; var entropy = 0; for (var i = 0; i < hexString.length; i++) { var char = hexString[i]; frequency[char] = (frequency[char] || 0) + 1; } for (var char in frequency) { var p = frequency[char] / hexString.length; entropy -= p * Math.log2(p); } return entropy; } |
Troubleshooting Common Issues
App Crashes When Hooking
If the app crashes, try:
- Hook selectively: Start with just one or two hooks to identify the problematic one
- Check Android version: Some crypto APIs changed between Android versions
- Use try-catch blocks: Wrap hooks in try-catch to prevent crashes
1 2 3 4 5 6 7 8 |
try { cipher.doFinal.overload('[B').implementation = function(input) { // Your hook code here }; } catch(e) { console.log("[!] Failed to hook Cipher.doFinal: " + e); } |
Missing Crypto Operations
If you’re not seeing expected crypto operations:
- Check native libraries: The app might be using native crypto
- Look for obfuscation: Class names might be obfuscated
- Hook earlier: Some operations happen during app initialization
Performance Issues
If the app becomes slow:
- Reduce logging: Only log what you really need
- Use conditional hooks: Only hook when certain conditions are met
- Buffer output: Collect data and output in batches
Taking It Further
Integration with Other Tools
Combine Frida tracing with:
- Static analysis: Compare with what tools like JADX show you
- Network monitoring: Use Burp Suite or mitmproxy alongside
- Binary analysis: Check native libraries with tools like Ghidra
Building a Crypto Testing Framework
You could build a complete framework:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
# crypto_analyzer.py class CryptoAnalyzer: def __init__(self, package_name): self.package = package_name self.findings = [] self.device = frida.get_usb_device() def analyze_keys(self): # Load key analysis script pass def analyze_algorithms(self): # Load algorithm analysis script pass def generate_report(self): # Generate comprehensive report pass # Usage analyzer = CryptoAnalyzer("com.example.app") analyzer.analyze_keys() analyzer.analyze_algorithms() report = analyzer.generate_report() |
Continuous Monitoring
Set up continuous monitoring for apps in development:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
#!/bin/bash # crypto_monitor.sh APP_PACKAGE="com.yourapp.debug" LOG_DIR="/tmp/crypto_logs" DATE=$(date +%Y%m%d_%H%M%S) mkdir -p $LOG_DIR echo "Starting crypto monitoring for $APP_PACKAGE" frida -U -l crypto_tracer.js $APP_PACKAGE > "$LOG_DIR/crypto_trace_$DATE.log" 2>&1 & FRIDA_PID=$! echo "Frida PID: $FRIDA_PID" # Monitor for 10 minutes sleep 600 kill $FRIDA_PID echo "Monitoring complete. Log saved to $LOG_DIR/crypto_trace_$DATE.log" # Analyze the log python analyze_crypto_log.py "$LOG_DIR/crypto_trace_$DATE.log" |
Wrapping Up
At this point, you should have a solid understanding of how to trace crypto operations in Android apps. This technique gives you incredible visibility into what’s really happening with sensitive data.
Remember, with great power comes great responsibility. Use these techniques ethically and only on apps you have permission to analyze. Whether you’re doing security research, debugging your own code, or just satisfying your curiosity, always respect privacy and follow responsible disclosure practices.
The crypto landscape is constantly evolving, and so should your analysis techniques. Keep experimenting, keep learning, and most importantly, keep making the mobile world a more secure place.
Now go forth and uncover those crypto secrets! And remember – if you find something interesting, the community always appreciates researchers who share their knowledge responsibly.
Great information shared.. really enjoyed reading this post thank you author for sharing this post .. appreciated
I do not even understand how I ended up here, but I assumed this publish used to be great
For the reason that the admin of this site is working, no uncertainty very quickly it will be renowned, due to its quality contents.